Say "hello" to the world
As you saw in the video, you can see a function as a block of code with a name that performs a service. When it comes to a main
function, the service it performs is actually the program itself! Cool, right? When a function is located inside a class, it is called a method. Since all Java code is located inside classes, you can use both terms (functions and methods) interchangeably. 😎
Now that you know about main
functions and starting a program, let's write one! The tradition when learning a language is to write a first program that outputs the Hello World! string.
Here is the Java code honoring this tradition:
package hello;
/** This is an implementation of the traditional "Hello world!" message
*
* @author The OpenClassrooms Education team
*
*/
public class HelloWorld {
/** This is where the program starts */
public static void main(String[] args) {
System.out.println("Hello World!");
}
}
Let's break this code down:
The first statement,
package hello;
, is a package declaration. A package defines a custom namespace for a related set of Java classes. In other words, you name a package and group together with related classes inside it. When writing a simple program, you can use the same package name for all your files.The
public class HelloWorld
declaration defines the name of the class asHelloWorld
. In Java, all code must reside inside a class. You will find yourself writing and using two kinds of classes:Classes that serve as a blueprint for objects, like you saw in the "Define objects" chapter. Those classes define the state of the object with fields, and its behavior with methods.
Utility classes that serve as containers for operations you want to perform, but do not need a persistent state. Those classes generally contain no fields.
Inside the class, you will find the
public static void main(String[] args)
function declaration. This is the piece of code that the Java interpreter looks for when you start a program.Inside the
main
method, you find theSystem.out.println("Hello World!");
statement that prints the expected message.In case you're curious, the lines within the
/**
and*/
characters are documentation comments. This creates a Javadoc, or in other words, an HTML web page containing the documentation for your code. It usually contains a list of classes, methods, and variables, as well as comments. This allows other programmers to use your code without having to go through your actual Java code. Handy, huh? For now, you'll be able to recognize what those lines do, but we'll come back to this at the end of the chapter. 😇
To sum up, the starting code for a Java program is contained inside a main function (or method). This main function is itself contained inside a class. Finally, this class itself belongs to a package.
Now that you know how to write the code, it's time to run it!
Run the program from Terminal
In Java, there is a direct mapping between:
Packages and folders
Classes and files
In other words, to run the program on your computer, create folders that correspond to your packages and files that correspond to our classes! For the moment, we have written our Hello World! code inside the main method of a HelloWorld class, which is in turn located inside a hello package. Now, let's check out what you have to do to map this with some files and folders.
There are a few key steps:
First, you've got to create a folder where you will put all of your code. This is typically called the root folder.
Inside this root folder, you can create a "hello" folder to match the name of your package.
Next, create a HelloWorld.java file inside the hello folder, to match the name of our class.
See how this all maps out? Package to folder, class to file. ✅ Once the HelloWorld.java file is created, you can type in your Java code. Let's use the code from the previous section:
package hello;
/** This is an implementation of the traditional "Hello world!" message
*
* @author The OpenClassrooms Education team
*
*/
public class HelloWorld {
/** This is where the program starts */
public static void main(String[] args) {
System.out.println("Hello World!");
}
}
When all the code is inside the file, you need to convert this Java code into a machine-executable code the computer can understand.
What does "machine-executable" mean?
Regardless of the programming language you are using to write your code, at the end of the day it must be translated to a set of instructions that a computer can run. This is called machine code.
Why don't we write programs directly in machine code?
Even though the machine code is very clear to computers, it would be very hard to utilize for humans. Think of it as a cooking recipe; you can use a spoken language to describe the ingredients and the instructions, or, you can use their chemical contents and chemistry jargon to describe the instructions. It may be understood by some, however, for most of us cooking would be out of reach. Even for those who understand, it would turn into a very long process! Don't you agree?
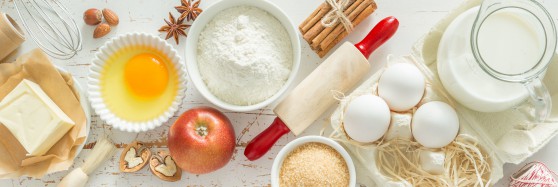
The language the Java code must be transformed into is called Bytecode. To transform Java code into Bytecode, use the javac compiler.
This is where folders start to become useful! Using Terminal, navigate to the root
folder of your program and run the following command:
$ javac hello.HelloWorld.java
This command creates a HelloWorld.class file inside the hello folder. This file is a binary file (you cannot open it in a text editor). You can now run the program with the java (or java.exe on windows) command:
$ java hello.HelloWorld Hello World!
Victory! ✌️In the remainder of this chapter, we will concentrate on how to organize your code so that your main
remains as small as possible.
Organize your code to keep your main as small as possible
The purpose of your main
function is to start your program. It actually is your program. If you were to provide all the statements the logic of your program requires inside the main
, it would quickly become impossible to read and understand. This would make it tough to maintain your program. This is why you need to organize your code into classes.
As we said at the beginning of the chapter, there are two kinds of classes you can write and use.
Classes as blueprints for objects
As seen in the first part of this course, you can define complex types that put together different attributes representing a named concept. Those are blueprint classes. You often write them to model the domain of your application - what you are writing your program to do.
An example of such a blueprint class is the String
class which you use to store and manipulate strings in your program. This class is available in the java.lang
package, which is available from any part of your code.
How is String
a class and not a primitive type such as int
or double
?
String
is a class not only because its name starts with an uppercase letter, but because it defines a state and a behavior:
1. Its state is the character strings you are storing. The actual value is set for each object when you instantiate it.
2. Its behavior is the set of methods the String
class defines that let you operate on that string you store.
Let's see this in action:
package stringDemo;
public class StringDemo {
public static void main(String[] args) {
// Declare and create a string
String shockingSentence="The Java String type actually is a class, not a simple type!";
// Say it out loud
System.out.println(shockingSentence.toUpperCase());
// Reverse it
System.out.println(shockingSentence.replace("simple","primitive"));
}
}
Let's compile and run this program:
$ javac stringDemo.StringDemo.java $ java stringDemo.StringDemo THE JAVA STRING TYPE ACTUALLY IS A CLASS, NOT A SIMPLE TYPE! The Java String type actually is a class, not a primitive type!
As you can see, the String
class provides you with free functionalities! You can just use them to manipulate the string as you see fit.
How can I guess what functionalities are actually available?
Remember Javadoc? Whenever you write a class, you are supposed to document it within the /**
and */
markers. Doing so helps generate an HTML documentation page automatically. The Java developers have done that for you and made it available on the official website. Take a look at the String
Javadoc page. Can you find the toUpperCase()
and the contain()
methods?
Another type of blueprint class we have already covered is the ArrayList
class. It offers all the functionalities you need from a list. You need to instantiate a new object for each list in your program. Each object will contain its own list elements.
Let's now see the second type of classes you will define and use: utility classes.
Utility classes as support for your code
You've also used the System
class. Remember? We just used it to print a string! Take a moment to explore its Javadoc. 😇 Did you notice the in
, out
, and err
static fields the System
class defined?
Let's break down the statement of our first program:
System.out.println("Hello World!");
System
is the name of a class.out
is a static field representing the standard output of your computer. In practice, that means the screen, unless you redefine it.println
is a static method that performs the printing operation and goes to a new line.
As both the out
field and the println
method are defined as "static," which means you don't need to instantiate an object to use them. This is why you are calling the method directly on the System
class.
Another example of a utility class is the Math
class.
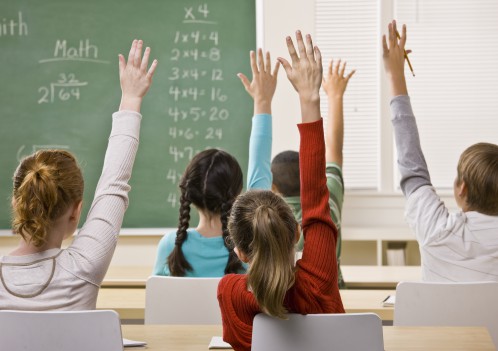
The Math
class defines common operations you can perform on numbers. For instance, Math.abs(-4);
will result in the absolute value of the provided value, which is 4
in that case.
Static methods defined into utility classes are useful because they let you extract a functionality into a reusable block of code. You can then use them easily without having to instantiate an object. This means you have no excuse for a lengthy main
!
Clean up your main
To complete this chapter, let's see how to apply a fundamental concept to make your code understandable and maintainable: do not keep anything inside the main
that can be extracted to a function. Even if your current implementation consists of a single statement, you should replace it with a call to an actual method that does the work. If you decide to add more complexity, it will be moved to other methods, keeping the main
clean.
Let's get control of your main
. That means we'll make it as clean as possible. Here is a clean implementation of our HelloWorld program:
package cleanHello;
/** This is a clean implementation of the traditional "Hello world!" message
*
* @author The OpenClassrooms Education team
*
*/
public class CleanWorld {
/** This is where the program starts */
public static void main(String[] args) {
sayHelloTo("world");
}
/** prints a hello message to the provided recipient
*
* @param recipient
*/
private static void sayHelloTo(String recipient) {
System.out.println("Hello " + recipient);
}
}
As you can see, the CleanWorld
class defines two methods:
main
is the starting point of the program. Its only job is to hand over the work to the sayHello method with the argument it needs. In our case, this is the predefined recipient to our hello: the world!The
sayHello
method prints the "Hello" string and appends the value provided to therecipient
variable whenever it is called by themain
method.
In terms of functionality, nothing has changed. However, you can now add more logic to the message you will display by changing the sayHello
method and personalizing the recipient name.
We'll add functionality in the following chapters! For that you need to add more logic to your Java programming tool belt. The next chapter covers the first tool: conditions!
Summary
In this chapter, you have seen that:
Java programs are structured into packages and classes.
No code is written outside of a class, which means that all functions are methods in Java.
Packages map to folders and classes to files.
The javac command converts Java code into Bytecode.
The java command runs the actual program by running the
main
function inside the provided class.Classes come in two flavors:
Blueprint classes that are used as models for the instantiation of objects.
Utility classes which contain static methods that can be called directly on the class.
Prefixing classes and methods with documentation comments
/**
and*/
allows you to generate an HTML page with all the documentation for the class, called a Javadoc.The
main
method can be hidden from you if you use a framework.Clean code principles mandate that that no logic is written inside the
main
method. All work should be delegated to properly named functions.