What is debugging?
De-bug(g)-ing- is as you can see, literally means to eliminate bugs.
And what are bugs?
They aren't the little critters running around outside! Bugs are mistakes in the logic of a program and are both algorithmic and business logic mistakes.
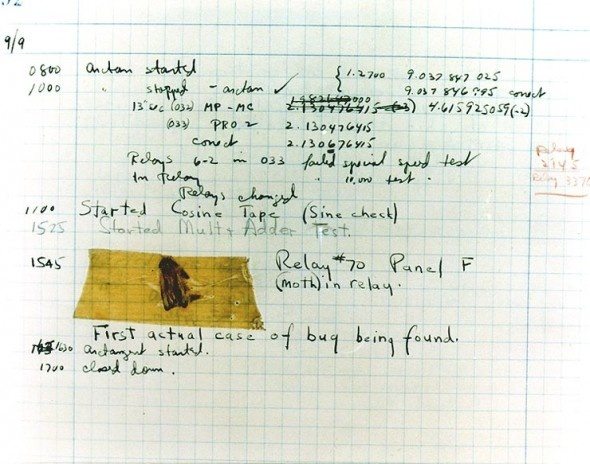
This is a cute story, however, software bugs are still pretty annoying. They are equally annoying if they were planted by you or other development participants 😉 (for different reasons I must note).
Bugs are not compilation errors - at least those are always right in your face, and you know where to find and fix them even if you don't always know how.
Let's reiterate. Bugs are either algorithmic or business logic mistakes. They may cause crashes or produce incorrect results. Incorrect results are often more dangerous than crashes as they may continue silently happening without an immediate effect. 😱
There are a number of approaches to detect and eliminate bugs:
Random checks - poking around
Using debugging tools
Random checks
This is the tried and true method which is effective on a small scale when you need to fix simple issues. And let's face it, you'll use it all the time for quick checks, and it's fine. The important part is not using it as a tool to ensure the code quality. The most common way of implementing this method is using print
function.
As an example, let's say you are developing a function that converts kilograms to pounds and then utilize it within the logic of your app:
class MagicWeight {
public static double kgToLb(double kg){
double k = 2.20462262185;
double lb = kg / k;
return lb;
}
}
double weightInKg = 50.0;
double weightInLb = MagicWeight.kgToLb(weightInKg);
At some point, using the result of this function, you may suspect that something is not quite right. So you'd want to verify the value this function returns.
The most popular way of doing a quick check is using a print
function. So let's print the result after we've called the function:
class MagicWeight {
public static double kgToLb(double kg) {
double lb = kg / k;
return lb;
}
}
double weightInKg = 50.0;
double weightInLb = MagicWeight.kgToLb(weightInKg)
System.out.println(weightInKg+ " kg equals "weightInLb+" lb"); // -> 50.0 kg equals 22.679618499987406 lb
Well, that doesn't look right 🤨.
Next, you may want to get closer to the calculation and print the values within the calculating function:
class MagicWeight {
public static double kgToLb(double kg) {
double lb = kg / 2.2;
System.out.println("Converted "+kg+" kg to pounds and the result is "+lb+" lb")
// -> Converting 50.0 kg to pounds and using conversion coefficient 2.20462262185 and getting the result 22.679618499987406 lb
return lb;
}
}
double weightInKg = 50.0;
double weightInLb = MagicWeight.kgToLb(weightInKg);
System.out.println(weightInKg+" kg equals "+weightInLb+" lb");
Here you can expose an additional value being used for the calculation -k
- just to make sure it contains the correct value. The new output also shows a suspicious result. This allow you to conclude that you must look for an error within the calculated function. Of course, it's using division instead of multiplication. Let's fix that!
class MagicWeight {
public static double kgToLb(double kg){
double k = 2.20462262185;
double lb = kg * k;
System.out.println("Converted \(kg) kg to pounds and the result is \(lb) lb");
// -> Converting 50.0 kg to pounds and using conversion coefficient 2.20462262185 and getting the result 110.23113109250001 lb
return lb;
}
}
double weightInKg = 50.0;
double weightInLb = MagicWeight.kgToLb(kg: weightInKg);
System.out.println(weightInKg+" kg equals "+weightInLb+" lb"); // -> 50.0 kg equals 110.23113109250001 lb
Now that looks like the right number!
You can see that having the values of variables printed during different steps of the instructions in the program helps you see the state of those values. You can then trace the mistake to its roots and fix it.
Using debugging tools
The debugging tools and processes you use will depend on the programming language, platform, and environment you are utilizing. However, these tools share many common concepts.
The development tools
The most common development environment for Java development is Eclipse - a free integrated development environment (IDE) available on the internet.
The process of debugging means going through the sequence of instructions step by step revealing the current state of the variables in scope. "Variables in scope" refers to the variables that are declared within the code block which you are currently inspecting (during debugging). And "revealing" means that you can see the values of these variables at that moment. As you can imagine, it's a more complete way of checking out more complex problems.
Summary
In this chapter, you learned the following:
Bugs are algorithmic and business logic mistakes.
Debugging is the process of detecting and eliminating bugs.
The most common ways to detect bugs are:
Random checks - good for quick validation
Using debugging tools - for a more targeted precise process