In this chapter, we will learn how to interact with the user and respond to their actions.
Managing Actions
The two key actions we need to implement are as follows:
Verify that the user entered their first name
Detect when the user presses the Play button
User Input
We have to guarantee that the user has entered their first name before they can proceed; not because we're evil, but for the sake of your education (but maybe we're also a little evil 😈). To prevent the user from going further, the easiest way is to disable the "Play" button at launch and activate it after the user enters their name.
To begin, add the following line to your onCreate()
method:
mPlayButton.setEnabled(false);
This line disables the button. Be sure to add this after connecting themPlayButton
variable, otherwise you will call setEnabled()
on a null object, and this will certainly crash the application.
After disabling the button, we must be notified when the user begins to type in the corresponding EditText
field. To do this, we will invoke the addTextChangedListener()
method on the EditText
instance, using an anonymous class as our interface implementation.
Add the following to your onCreate()
method:
mNameInput.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
// This is where we'll check the user input
}
@Override
public void afterTextChanged(Editable s) {
}
});
To avoid excessive typing (and carpal tunnel), Android Studio helps you yet again. First, AS will offer suggestions when you begin to type "addText." You can press the tab key to complete the method. Second, as you add the parameter, stop after typing "new T." Android Studio will suggest you automatically implement the associated interface.
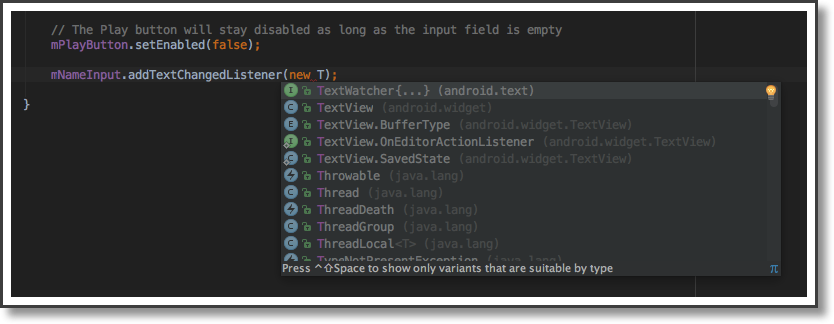
The only method of interest to us is onTextChanged()
.
Each time the user enters a letter, EditText will call the onTextChanged()
method of our interface. This allows us to determine whether the user has entered their first name, in which case we want to activate the Play button.
Here's the logic we'll use: if the user enters at least one letter, then we enable the button (yeah, single letter first names are quite rare, but you never know which James Bond supporting character will show up to play TopQuiz: M, Q... Z? 🤵).
Adding the following line should do the trick:
mPlayButton.setEnabled(s.toString().length() != 0);
Click the Button
Once the button is enabled, the user can click on it to start the game (we will see this in the next chapter). To detect a click, we have to implement a listener.
The principle is identical to handling user input as above. Call the setOnClickListener()
method on the mPlayButton
object, and then implement View's OnClickListener interface, like so:
mPlayButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// The user just clicked
}
});
The onClick()
method will receive an invocation every time the user clicks the button. This is the ideal place from which to start the game, which we will implement in the next chapter.
Let's Review With a Demo
You can see these steps in the video below:
Let's Recap!
You can manage the attributes of elements in the Activity to make them react to user actions.
To detect a button click, implement a
View.OnClickListener
interface.Use the
afterTextChanged()
method to detect a change in the text of an EditText.
Now you know how to manage user actions. Guess what’s next? Launching the app on an emulator or a real device!