In this chapter, we will study how to backup information locally, such as the user's score and their first name. So when the player restarts the application, we can greet them and help them improve their high score.
Managing Preferences
SharedPreferences is the API that Android provides to backup small pieces of local information. It is an abstraction layer that makes life easier: it retrieves and stores your data in an XML file.
The API also lets us spread the data across multiple files. That feature is useful in applications with varied data. We will use the standard version for now: a single storage file that Android creates and manages automatically.
To access an instance of SharedPreferences, call the getPreferences()
method in the current Activity, like so:
SharedPreferences preferences = getPreferences(MODE_PRIVATE);
MODE_PRIVATE specifies that the data within the file may only be accessed by our application. In earlier versions of Android, other modes allowed third-party applications to read and write your preference files. For security reasons, Google decided to make those modes obsolete, so remember to stick to MODE_PRIVATE.
Writing Preferences
To modify the information stored in SharedPreferences, we must use the SharedPreferences.Editor API.
Stored information is always associated with a key, this enables precise retrieval of desired values without having to read all the data.
We must specify the type of data stored by using the appropriate method. For example, to store a string, you will need to invoke Editor.putString()
. To store an integer, we useEditor.putInt()
.
To commit a change to storage, we have to call Editor.apply()
.
For example, here's how we can store the user's first name:
SharedPreferences preferences = getPreferences(MODE_PRIVATE);
SharedPreferences.Editor editor = preferences.edit();
editor.putString("firstname", mUser.getFirstName());
editor.apply();
You may have noted the following two things:
The key used to store the user's first name is, "firstname"
The user's first name is retrieved from the model
Creating a constant for "firstname" is best practice. The code will be easier to maintain and less subject to typing errors.
Reading Preferences
As with writing, reading a value requires calling a specific method depending on the data type. For example, to retrieve a string, use Editor.getString
() , and retrieve an integer with Editor.getInt()
. For example, to retrieve the user's first name, use the following code:
String firstname = getPreferences(MODE_PRIVATE).getString("firstname", null);
Note the second parameter, null: it allows us to specify a default value in case the requested value does not exist. This makes sense in some applications.
In ours, if the first name has never been stored, then there is no need to return a default one. We therefore return null, which lets us know that this is the first time the user has launched TopQuiz.
Let's Review With a Demo
You can see these steps in the video below:
Implementation
In the previous section, you stored and retrieved the user's first name, all in MainActivity.
Exercise
Well, time to give you some work to do. Update MainActivity as follows:
When the Activity starts, check if the user has already played the game
How would you go about doing this?
Retrieve the preference values and update the welcome text
The text should greet the person and remind them of their most-recent score
Add the player's first name into the input field
Place the cursor at the end of their name
And update the text after a game ends
Print their most recent score
The result should look like this:
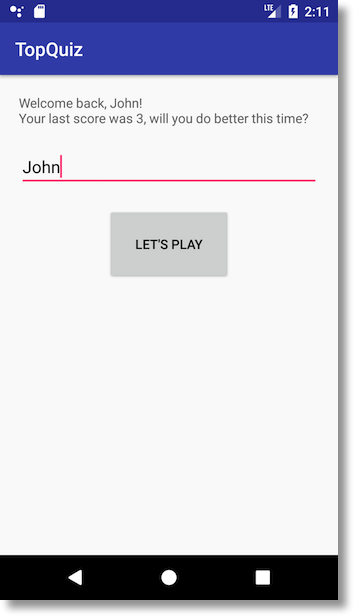
Let's Recap!
You can save information locally using the SharedPreferences API.
To access an instance of SharedPreferences, call the getSharedPreferences() method in any Activity.
Use the SharedPreferences.Editor API.
Now you can back up simple data with SharedPreferences! In the next chapter, we’ll see how to use SharedPreferences to improve the user experience.