Why write well structured code?
It’s okay, we’ve all done it.
Made a mess of our CSS, that is. It’s not that it starts in disarray, but rather it’s something that happens incrementally, change by change. And, unless you’re prepared and vigilant, you’ll end up with a CSS code base in chaos before you realize it’s happening.
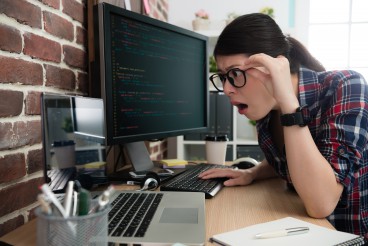
Is this really such a big deal? If you get the project done, what's the problem?
To answer that question, let’s take a trip through the life of a theoretical website:
A client tasks you with creating a contact form, involving just a few text inputs and a button. You write the HTML markup and style it, assigning a class name of btn
to the button, which has the following rule set, or group of CSS instructions:
.btn{
background-color: #001534;
color: #15DEA5;
border-radius: 3rem;
width: 100%;
padding: 1.5rem;
}
It looks good, and the client is happy. Indeed, so happy that they ask you expand their site. Suddenly, you need a bunch of different buttons of different sizes, colors, and shapes. And since .btn
is pretty specific, you end up with classes like .btn-alt
, .btn-big
, and .btn-round
. Later you add .btn-small
and .btn-alt-2
. Are you starting to see the problem?
I can just go in and change the names again manually, right? There aren’t that many. And if the client likes it, what does it matter?
Okay, sure, it looks good, and the client is even happier - for a while. But eventually they need a few bits updated, a few things added on, and you have to dive back into your code. And that's when you have to start asking yourself: What does .btn-alt
look like, again? How is.btn-alt-2
different? And just how big is.btn-big
?
Rather than tapping out your changes quickly and easily, you find yourself spending a disproportionate amount of time wading through the spaghetti that your CSS has become. How are you supposed to keep track of all your different elements, especially in a large project? How do you manage CSS that you’re constantly adding on to and updating?
CSS is notoriously tricky to keep in line. It lacks a lot of the structure you find in most other programming languages. You can see these even by comparing some sample code in HTML and CSS.
HTML
<body>
<main class="container">
<form class="form">
<h1 class="form__heading">Contact me</h1>
<div class="form__field">
<label for="contact-name">name</label>
<input type="text" name="contact-name" id="contact-name">
</div>
<div class="form__field">
<label for="contact-email">email</label>
<input type="email" name="contact-email" id="contact-email">
</div>
<div class="form__field">
<label for="mssg">message</label>
<textarea name="mssg" id="mssg" cols="30" rows="10"></textarea>
</div>
<button class="btn btn--full-width">Submit</button>
</form>
</main>
</body>
CSS
.container {
margin: 6rem;
padding: 1.5rem 1.5rem 0 1.5rem;
border: 0.1rem solid #D6FFF5; }
}
.form {
width: 100%;
padding-bottom: 1.5rem;
}
.form__field label {
color: #D6FFF5;
display: block;
font-size: 2rem;
line-height: 2rem;
padding-top: 1.5rem;
}
.form__field input {
width: 100%;
background: #001534;
border: 0.1rem solid #15DEA5;
padding: 1.5rem;
color: #D6FFF5;
}
Notice that you can tell how the HTML elements above relate to one another by their hierarchy? While the CSS really just looks like a big, long list. There’s not much to indicate the relationships between the selectors, or their specificity. Keeping things in order when there isn’t much apparent structure to begin with, only makes things tougher. But we’re going to remedy that.
In this course, you’re going to learn how to write CSS that is both clean and maintainable by:
Implementing well-defined file structures.
Working with a CSS precompiler called SASS, a technology that improves your codebase and makes your life easier.
The you from the future says "thank you." 😉
Good fences make good neighbors
How should you go about writing your CSS to make sure that it’s clean and maintainable? Step one is finding categories. Go ahead and scroll through your CSS or the chunk of CSS from the previous section. You will likely begin to notice heaps of repeated rules. For example, all of your buttons are using the same padding and font color, and nearly all of them have the same background color. This means that you have repeated your code.
There’s a concept in programming known as the DRY principle, which goes like this: Don’t repeat yourself. Those piles of repeated CSS rules are preventing you from achieving a DRY codebase, and are wreaking havoc on its maintainability by making it cumbersome to update values. The more times you repeat your padding rule, the greater the odds of missing a value when you update the padding from, say, 2rem to 3rem.
Okay, so what do I do now? 🤔
Begin by refactoring, or in other words, cleaning up your code. Start by looking through all of your selectors and finding commonalities. Let’s take the example of a button. Check out all of the buttons described in the code and make note of which rules are the same. Let’s say that you notice that all your buttons have the same background color, color, and padding. You might end up with something like:
.btn {
background-color: #001534;
color: #15DEA5;
padding: 1.5rem;
}
Without those other rules, the button will only be the width of its contents, plus padding. And the corners. They’re square!
Not to worry, any surplus rules from the original .btn
are split into new, more specific selectors:
.btn-wide {
width: 100%;
}
/* And... */
.btn-rounded {
border-radius: 3rem;
}
So instead of your HTML looking like this:
< button class="btn" >...</button>
Your markup looks like:
< button class="btn btn-rounded btn-wide" >...</button>
What we’ve just done is called separation of concerns. In the .btn
selector, we’ve defined the very essence of our buttons, and nothing more. One selector = one set of basic rules. Every button in your code will have these qualities. In the .btn-wide
and .btn-rounded
selectors, we’ve added additional rules that, when combined with .btn
, will modify the button.
By separating concerns, your codebase instantly becomes easier to maintain; instead of relying onCtrl+Fand Find-and-Replace to update your buttons’ padding, you only need to change the value on a single rule set, and it will cascade through the rest of your site.
Again, future you sends their gratitude. 🙌
Have a plan and stick to it
There are no hard, set-fast rules for architecting your CSS - only educated opinions. A quick bit of googling would serve you up heaps of medium.com entries on the subject, some in agreement, others not so much, while others consent, but with provisos. Amidst all this controversy, we've chosen to present you with practical solutions. In other words, the means and methods of this course are battle-tested, highly adopted practices within the industry, whose foundations will serve you well in creating a clean, maintainable CSS codebase on projects of all scales.
The most important takeaway in building a strong, maintainable front-end code is to sit down, make a plan, and stick with it. Instead of looking through your existing code like we did in the example above, you need to think about how to structure your code before you even write a line.
Coming up, we’ll start to do just that, by showing you how to implement a well-defined, highly-semantic naming convention for your selectors. But in order to do that, you first need to understand how browsers decide which styles to apply to your elements. Luckily for you, that's what the next chapter is about!
Try it out for yourself!
Your turn to code in this interactive exercise on CodePen! Let’s a look at a heading class and separate its concerns. Right now it has all of the properties that create the styling of our headings, as well as a property to convert the text to uppercase. The problem is that we don’t always want to capitalize our headings.
Instead of making a whole-new, non-capitalized heading, let’s move .heading
’s text-transform property into its own class, called .heading-caps
. Then we can add it to headings where we want it to be in uppercase.
Create a new class called
.heading-caps
Move the text-transform property into
.heading-caps
In the HTML, add
heading-caps
to the classes of the second heading
You should end up with the first heading in lowercase, while the second heading stays uppercase! Check your work in this CodePen.
Let's recap!
CSS doesn’t have a rigid structure, which can make it hard to keep a clean and maintainable codebase.
By creating and implementing your own structure, you can keep keep an unwieldy CSS in check.
When you create selectors, it’s important to not cram too many rules into it. Separating concerns helps to keep your code more readable and maintainable.