In the last chapter, you learned how to set column and row sizing for your grids. While we've only worked with squares of different colors so far, the same principles can be applied to real websites to organize content!
We'll continue working with these squares in order to focus on the grid concepts themselves. Here's where we left off in the last chapter:
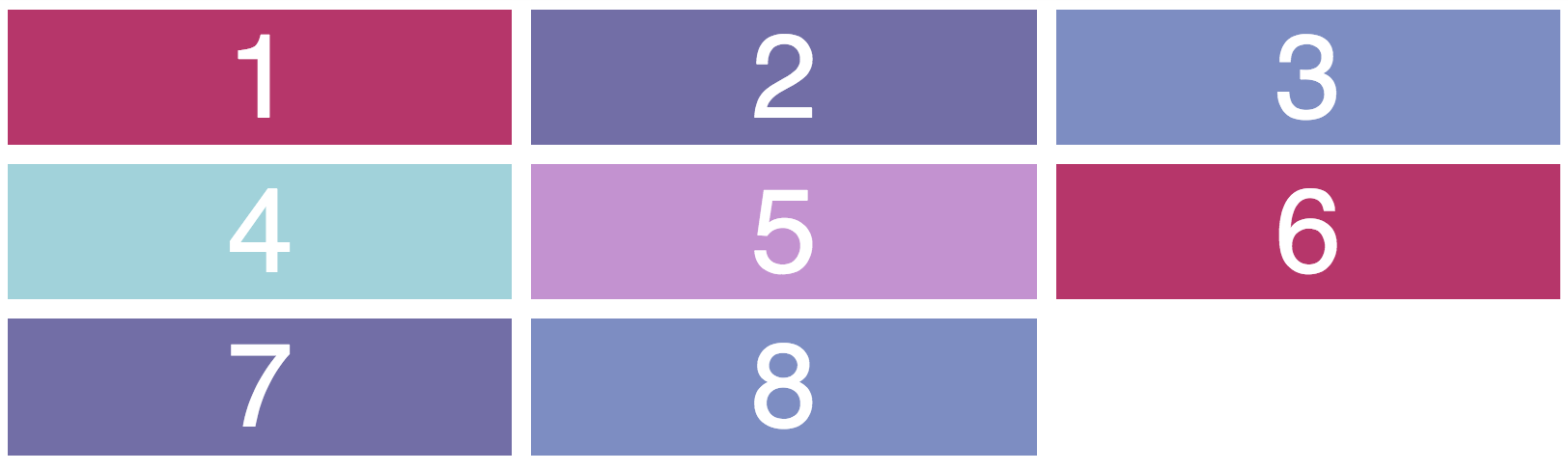
HTML
<div class="container">
<div>1</div>
<div>2</div>
<div>3</div>
<div>4</div>
<div>5</div>
<div>6</div>
<div>7</div>
<div>8</div>
</div>
CSS
.container {
display: grid;
grid-template-columns: 1fr 1fr 1fr;
grid-auto-rows: 1.6em;
grid-gap: 10px;
}
We'll cover two shorthand ways to write the code above. The result will be the same!
The repeat function
When setting grids, you'll often have values that repeat. In our above example, there are 3 columns that are each 1fr wide. You can create the 3 columns by specifying each of their widths one-by-one, as you've already seen, or you can use a CSS function called repeat to create a repeating pattern.
Inside the repeat function, you must specify:
the number of times you want the measurement pattern to repeat
the measurement(s) in pixels, em, percentages, fr...whichever unit you're feeling
In our example, we want 3 columns, each 1fr wide.
Therefore,
grid-template-columns: 1fr 1fr 1fr;
can also be written as:
grid-template-columns: repeat (3, 1fr);
Translated into ordinary language, this reads as "Repeat a 1fr measurement 3 times." The visual result is exactly the same.
Using repeat, you can even create repeating patterns with multiple measurements! To create 2 columns, one that's 0.5fr wide, and another that's 1fr wide, and then to repeat that pattern twice, you could write the following line of code:
grid-template-columns: repeat(2, 0.5fr 1fr);
Translated into ordinary language, this reads as "Repeat a pattern with 0.5fr and 1fr columns 2 times." The result is:
Column 1 is 0.5fr wide.
Column 2 is 1fr wide.
Column 3 is 0.5fr wide.
Column 4 is 1fr wide.
Row and column specification shorthand
You've previously defined grid measurements using separate lines of code to describe rows and columns.
Here's our example written two separate ways (one with the repeat function and the other without):
.container {
grid-template-columns: 1fr 1fr 1fr;
grid-template-rows: 1.6em 1.6em 1.6em;
/* or using the repeat function:*/
grid-template-columns: repeat(3, 1fr);
grid-template-rows: repeat(3, 1.6em);
}
The separate column and row measurements can be combined into one line of code. The new property is simply called grid-template — the same as the properties you already learned, just without -column or -row at the end!
Within the grid-template property, you'll:
specify your row measurements
type a forward slash
specify your column measurements
You must provide specifications in that order!
Let's apply this shorthand syntax to our multicolor grid example:
.container {
grid-template: 1.6em 1.6em 1.6em / 1fr 1fr 1fr;
/* or using the repeat function:*/
grid-template: repeat(3, 1.6em) / repeat(3, 1fr);
}
The result is the same, except you used one line of code instead of two. Efficient!
Don't forget to create a default row height separately using the grid-auto-rows property if you think additional rows may pop up someday. This can't be combined into the grid-template shorthand notation and must be written on a separate line.
Your Turn
In the following interactive exercise, you'll rewrite some grid code using the repeat function and grid-template shorthand. In the next chapter, we'll check out how to set measurements for each element itself within the grid.
Find the style.css
file, which is inside the css
folder.
Locate the style rules for .container
. You'll see a set of rules for grid-template-columns
and grid-template-rows
.
Combine the column and row specifications into one line of code using grid-template
. Remember: the row specifications come first, followed a forward slash, followed by the column specifications. And check out your results!
Recap
Use the
repeat
function to make a series of rows or columns of equal size. `Specify the number of repeats and the measurement to repeat within the function.
A repeat function for both rows and columns can be specified on the same line if you add a slash between the two repeat functions.